Distributed loads on frame elements in OpenSees are defined with respect to the local element axes as opposed to global axes. This choice made the implementation easy, but it can give OpenSees users more shadow work, like bagging your own groceries or pumping your own gas.
When global distributed loads act on inclined elements, e.g., snow loads on pitched roofs, you can’t simply apply a sine and cosine to transform the loads to local axes. The correct resolution onto local member axes requires a few steps that you learned in structural analysis. But, trust me, these steps are easily forgotten.
Given a global distributed load, you first compute the resultant load based on the projected length. Then, the resultant load is resolved into local components and finally, those components are spread along the element length.
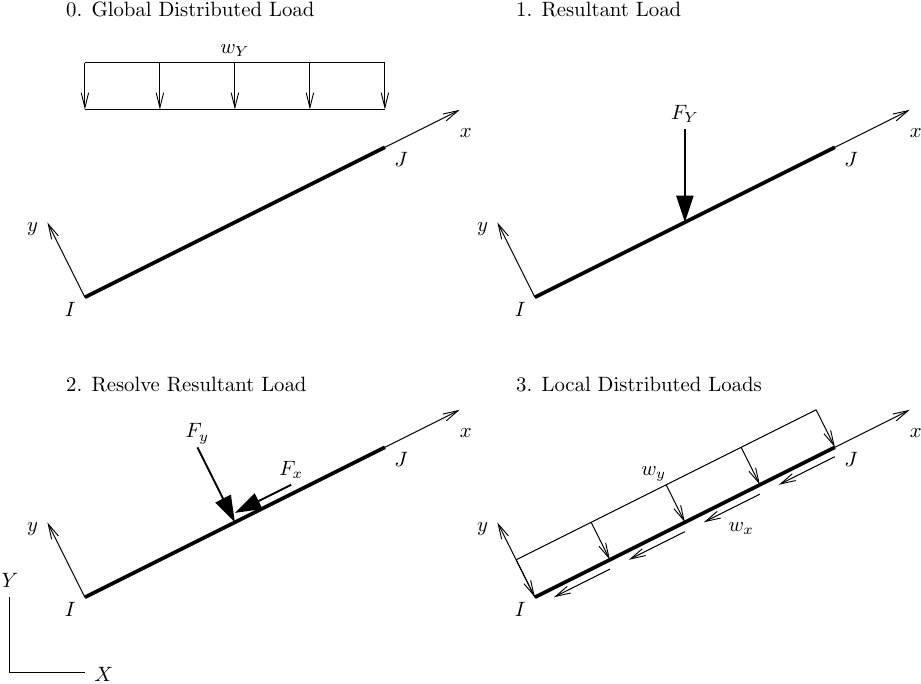
Here is OpenSees Python code to perform the above steps for 2D frame elements.
# For example, element tag 12
ndI,ndJ = ops.eleNodes(12)
dXY = np.subtract(ops.nodeCoord(ndJ),ops.nodeCoord(ndI))
L = np.linalg.norm(dXY)
# Change the index to 1 if global load in X-direction
LX = abs(dXY[0])
FY = wY*LX
xaxis = dXY/L
yaxis = [-xaxis[1], xaxis[0]]
# Change the index to 0 if global load in X-direction
# or use dot products if you feel sporty
Fx = FY*xaxis[1]
Fy = FY*yaxis[1]
wx = Fx/L
wy = Fy/L
The recent addition of local axes as recorder/eleResponse
options (PR #421) facilitates these steps for 3D frame elements.
# For example, element tag 12
ndI,ndJ = ops.eleNodes(12)
dXYZ = np.subtract(ops.nodeCoord(ndJ),ops.nodeCoord(ndI))
L = np.linalg.norm(dXYZ)
# Change the indices to 1,2 or 0,1 if global load in X- or Z- direction
LXZ = np.linalg.norm([dXYZ[0],dXYZ[2]])
FY = wY*LXZ
xaxis = ops.eleResponse(12,'xaxis')
yaxis = ops.eleResponse(12,'yaxis')
zaxis = ops.eleResponse(12,'zaxis')
# Change the index to 0 or 2 if global load in X- or Z- direction
# or use dot products if you feel sporty
Fx = FY*xaxis[1]
Fy = FY*yaxis[1]
Fz = FY*zaxis[1]
wx = Fx/L
wy = Fy/L
wz = Fz/L
After you get wx
, wy
, and wz
, you can apply them to an element in a load pattern using the eleLoad
command. It would also be straightforward to put these steps in a function.
Yes, setting distributed loads on frame elements in OpenSees is quite error prone. I will put these codes in a function for further use. Thank you, sir.
LikeLike
Hi P.D.
Can you provide a video for using pyopensees?
Best,
LikeLike
This one is pretty good 🙂
LikeLike
Dear Prof. Scott,
I hope you are well.
I have a question about span loads in OpenSees… The implementation of these loads in a linear or corotational transformation is:
pl(0) += p0(0);
pl(1) += p0(1);
pl(4) += p0(2);
where pl is the vector of forces containing the resisting forces at the local reference system and p0 are the equivalent end loads due to the span loads.
My question is that for a distributed load, I do not know how and where OpenSees considers the equivalent moment at the end of the element.
At the local level, we should see an equivalent moment due to the distributed load, where does OpenSees consider this moment?
Thank you in advance for your time and patience.
Kind regards,
Jose Baena.
LikeLike
I apologized… I found the answer in Neuenhofer and Filippou [1997].
Kind regards, Jose.
LikeLike
Hi Michael and thank you so much for this blog!
My question is: the command eleLoad also apply for plane shell elements or just for frames?
Thanks in advance!
Felipe Cordero.
LikeLike
This question is frequently asked on the OpenSees message board and Facebook group. The short answer is no, but there are other ways to apply surface loads to shells. If you would like more information, please get in touch.
LikeLike
Thanks Michael. I reviewed and it is what I supposed. So, by now, the best approach is calculating de nodal loads from the surface area. I know how to implement, and after looking at Facebook group and in the message board, there isn’t another way.
Thanks again.
LikeLike